目次
CNNモデル(評価・調整)
🚀 次のステップ
ここでは、CNN + LSTM モデルの評価 を行い、モデルの性能を詳細に分析します。
📌 目的
- Confusion Matrix(混同行列) の可視化
- Precision(適合率)、Recall(再現率)、F1-score の計算
- モデルの課題を特定し、次の改善案を考える
🛠 05_04_model_evaluation_CNN_LSTM.ipynb のコード
以下のコードを 05_04_model_evaluation_CNN_LSTM.ipynb
に追加して実行してください。
import os
import numpy as np
import tensorflow as tf
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.metrics import confusion_matrix, classification_report
from tensorflow.keras.models import load_model
from tensorflow.keras.utils import to_categorical
# 🚀 データ保存先
DATA_DIR = "./data/"
# 🚀 モデルのロード
model_path = os.path.join(DATA_DIR, "cnn_lstm_model_v2.keras") # 最新のモデルをロード
model = load_model(model_path)
# 🚀 テストデータのロード
test_features_path = os.path.join(DATA_DIR, "processed_features.npy")
test_labels_path = os.path.join(DATA_DIR, "processed_labels.npy")
X_test = np.load(test_features_path)
y_test = np.load(test_labels_path)
# 🚀 ラベルを one-hot encoding 形式に変換
if len(y_test.shape) == 1:
y_test = to_categorical(y_test)
# 🚀 データの正規化
X_test = X_test.reshape((X_test.shape[0], X_test.shape[1], 1))
# 🚀 モデルの評価
loss, accuracy = model.evaluate(X_test, y_test)
print(f"Test Loss: {loss:.4f}")
print(f"Test Accuracy: {accuracy:.4f}")
# 🚀 予測を取得
y_pred = model.predict(X_test)
y_pred_classes = np.argmax(y_pred, axis=1)
y_true_classes = np.argmax(y_test, axis=1)
# 🚀 Confusion Matrix の作成
conf_matrix = confusion_matrix(y_true_classes, y_pred_classes)
# 🚀 混同行列の可視化
plt.figure(figsize=(10, 8))
sns.heatmap(conf_matrix, annot=True, fmt="d", cmap="Blues", xticklabels=range(y_test.shape[1]), yticklabels=range(y_test.shape[1]))
plt.xlabel("Predicted Label")
plt.ylabel("True Label")
plt.title("Confusion Matrix")
plt.show()
# 🚀 Classification Report の出力(Precision, Recall, F1-score)
report = classification_report(y_true_classes, y_pred_classes, digits=4)
print("Classification Report:\n", report)
🔍 期待する結果
- Confusion Matrix
- どのクラスが正しく分類されているか?
- どのクラスで誤分類が多いか?
- Classification Report
- Precision(適合率) → モデルがどれだけ正確に予測しているか
- Recall(再現率) → 実際のクラスをどれだけ正しく識別できたか
- F1-score → Precision と Recall のバランス
- Test Accuracy の最終確認
- 50% を超えているか?
- クラスごとのばらつきがあるか?
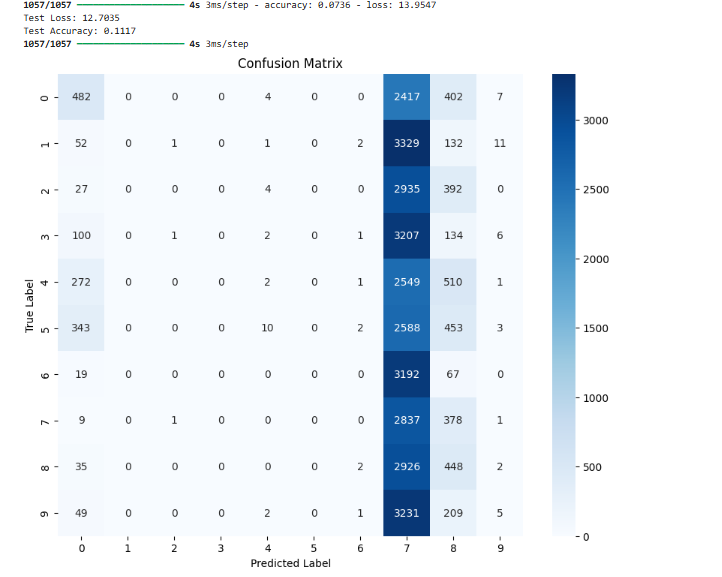
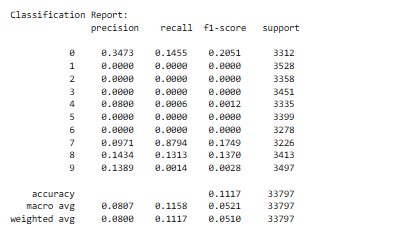
📌 評価結果の分析
❌ Test Accuracy: 11.17%(大幅な精度低下)
- テスト精度が極端に低下 → モデルがほぼ正しく分類できていない
- Loss が異常に高い(12.7035) → 学習が破綻している可能性が高い
- Confusion Matrix で ほぼ特定のクラス(7番)に偏っている
🔍 何が問題か?
- モデルが特定のクラス(7番)にほぼすべてのデータを分類してしまっている
- クラス 7 のサンプル数が圧倒的に多い?
- クラス不均衡が原因で、モデルが学習できていない可能性
- 学習データのラベルバランスを確認する必要あり
- Precision, Recall, F1-score の数値が全体的に低い
- クラス
1, 2, 3, 4, 5, 6, 8, 9
の予測がほぼゼロ - モデルが全く学習できていない
- クラス
- Loss 値が高すぎる
- 12.7 という異常な値 → 学習が失敗している
- 学習率の調整が必要
- バッチサイズ、正則化の見直しが必要
データの共通分割処理が手順から漏れていた可能性
確かに、モデルの学習前に 共通のデータ分割を行う Jupyter Notebook(例: 02_data_splitting.ipynb
) が適切に処理されていなかった可能性があります。一旦、学習をデータ分割あたりまで戻して段取り再検討。